JSP가 뭘까?
코드를 보며 설명하겠다.
JSP 코드
<head>
<title>lecture 4 - Email List application</title>
</head>
<body>
<%
String firstName = request.getParameter("firstName");
String lastName = request.getParameter("lastName");
%>
<h1>Thanks for joining our email list</h1>
<p>Here is the information that you entered:</p>
<table cellspacing="5" cellpadding="5" border="1">
<tr>
<td align="right">First name:</td>
<td><%= firstName %></td>
</tr>
<tr>
<td align="right">Last name:</td>
<td><%= lastName %></td>
</tr>
</table>
위 코드를 보면 마치 HTML 코드를 보는것 같다.
하지만 다르다.
여기 ~~.jsp 에 작성된 문법들은 객체화 되어 Servlet으로 들어간다고 생각하면된다.
어떤느낌인지 알기 위해 서블릿 코드를 보자
public class EmailServlet extends HttpServlet{
public void doGet(HttpServletRequest request,
HttpServletResponse response)
throws IOException, ServletException{
response.setContentType("text/html");
PrintWriter out = response.getWriter();
String firstName = request.getParameter("firstName");
String lastName = request.getParameter("lastName");
out.println(
"<html>\n"
+ "<head>\n"
+ " <title>lecture 5 - Email List application </title>\n"
+ "</head>\n"
+ "<body>\n"
+ "<h1>Thanks for joining our email list</h1>\n"
+ "<p>
Here is the information that you entered:
</p>\n"
+ " <table cellspacing=\"5\"
cellpadding=\"5\"
border=\"1\">\n"
+ " <tr><td align=\"right\">First name:</td>\n"
+ " <td>" + firstName + "</td>\n"
+ " </tr>\n"
+ " <tr><td align=\"right\">Last name:</td>\n"
+ " <td>" + lastName + "</td>\n"
+ " </tr>\n"
+ " </table>\n"
+ "</html>);
내용은 중요하지 않고 out.println에 쓰여진 코드를 보면 HTML 코드이다.
하지만 이 코드들을 이렇게 적으면 너무 힘들다.
그렇기 때문에 jsp를 쓴다.
위의 Servlet 코드를 보면
HttpServletRequest request, HttpServletResponse response 를 매개변수로 받고있는데
그게 이전에 설명한, 요청과 응답이다.
request 안에 클라이언트의 요청이 담겨있고
그 Data들을 꺼내서
로직을 생성하고
response 안에 클라이언트에게 응답할 내용을 담아서
다시 클라이언트에게 보내주는거
그게 Servlet의 역활이다.
Model 1 아키텍쳐
우리가 웹 페이지를 만들때 쓰이는 일정한 패턴이 있는데 가장 기초적이고(만들기 귀찮은) 패턴이 Model1 이다.
그래도 덧셈을 모르고 곱셈을 배우면 어렵운법....
실습을 통해 Model1 을 알아보자.
Model 1 아키텍처
- –제한된 요구사항의 웹 애플리케이션을 개발할 때 Model 1 아키텍처를 주로 사용한다.
- –Model 1 아키텍처에서의 비즈니스 클래스는 자바빈즈(JavaBeans)로 작성된다.
비즈니스 클래스는 애플리케이션의 비즈니스 프로세스와 데이터를 표현한다. - –데이터베이스나 파일 시스템을 데이터 저장소(data store)로 활용한다. 애플리케이션이
종료된 후에도 데이터는 유지되어야 하기 때문에 영구적인 데이터 저장소(persistent data storage)라고도 불린다. - –DAO 클래스와 같은 데이터 클래스의 메소드는 데이터 저장소의 데이터를 조회하거나 저장하는데 사용된다.
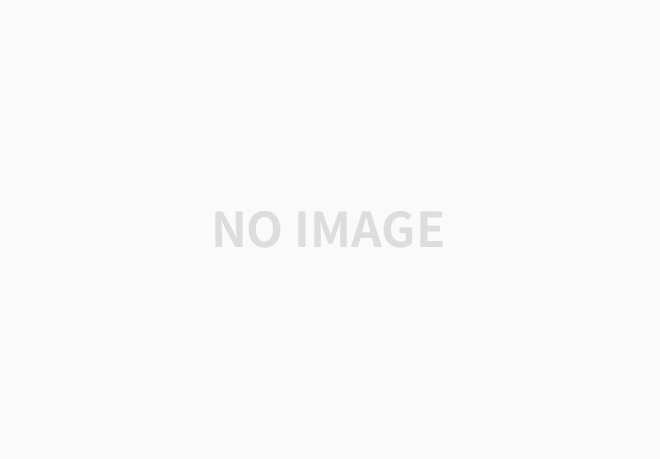
정말 간단해서 설명 할게 없고
실습할 emaillist 불러오기 구조를 간단하게 그려봤다.
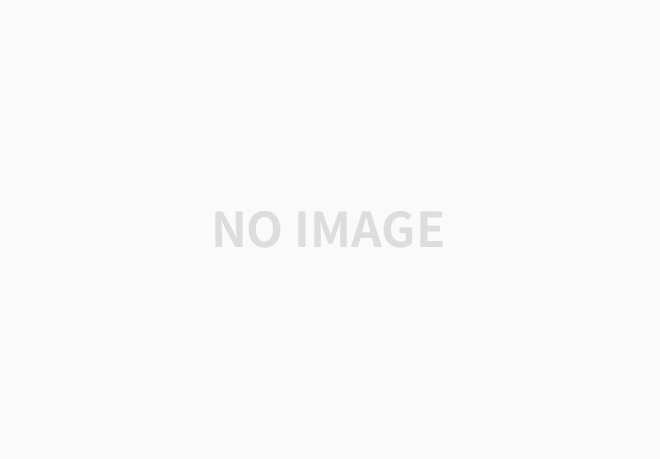
위의 아키텍쳐 구성과 비교해서 잘 보면 된다.
https://github.com/stpn94/servlet-practices/tree/master/emaillist01/src/main
전체 코드는 위의 링크에 들어가면 있다.
클라이언트가
어떤 홈페이지 화면에서
성 : 이
이름 : 종윤
이메일 : good@good.com
추가
추가 버튼을 누르면
form.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<h1>메일 리스트 가입</h1>
<p>
메일 리스트에 가입하려면,<br>
아래 항목을 기입하고 submit 버튼을 클릭하세요.
</p>
<form action="<%=request.getContextPath() %>/add.jsp" method="post">
First name: <input type="text" name="fn" value="" ><br>
Last name: <input type="text" name="ln" value=""><br>
Email address: <input type="text" name="email" value=""><br>
<input type="submit" value="등록">
</form>
<br>
<p>
<a href="<%=request.getContextPath() %>">리스트 바로가기</a>
</p>
</body>
</html>
위의 from.jsp를 실행시킨거와 같다.
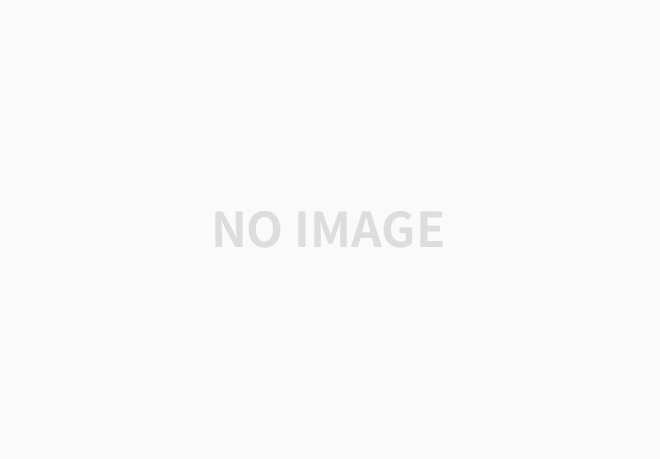
그럼
add.jsp 로 보내줄꺼고
add.jsp에서
<%@page import="com.douzone.emaillist.dao.EmaillistDao"%>
<%@page import="com.douzone.emaillist.vo.EmaillistVo"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
request.setCharacterEncoding("utf-8");
String firstName = request.getParameter("fn");
String lastName = request.getParameter("ln");
String email = request.getParameter("email");
EmaillistVo vo = new EmaillistVo();
vo.setFirstName(firstName);
vo.setLastName(lastName);
vo.setEmail(email);
new EmaillistDao().insert(vo);
response.sendRedirect(request.getContextPath());
%>
여기서 EmaillistDao()를 실행시켜서
DB에 이 종윤의 Data를 입력 시켰다.
그리고 index.jsp로 리다이렉트 해준다.
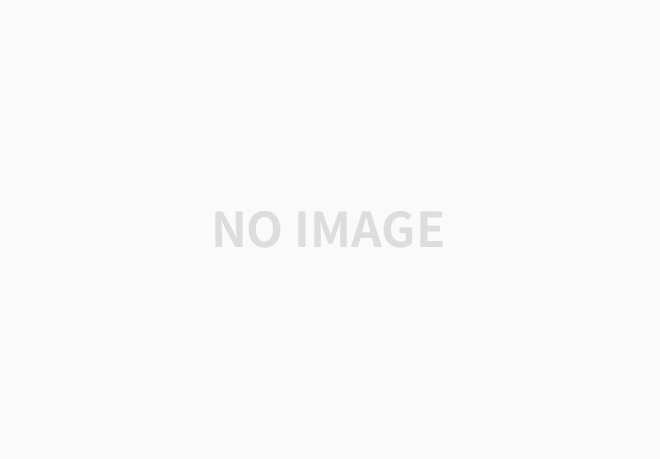
클라이언트가
이메일을 보고싶다고
요청을 보내면
index.jsp 로 가서
<%@ page import="com.douzone.emaillist.vo.EmaillistVo"%>
<%@ page import="java.util.List"%>
<%@ page import="com.douzone.emaillist.dao.EmaillistDao"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
List<EmaillistVo> list = new EmaillistDao().findAll();
%>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<h1>메일 리스트에 가입되었습니다.</h1>
<p>입력한 정보 내역입니다.</p>
<%
for (EmaillistVo vo : list) {
%>
<table border="1" cellpadding="5" cellspacing="2">
<tr>
<td align=right>First name:</td>
<td><%=vo.getFirstName()%></td>
</tr>
<tr>
<td align=right width="110">Last name:</td>
<td width="110"><%=vo.getLastName()%></td>
</tr>
<tr>
<td align=right>Email address:</td>
<td><%=vo.getEmail()%></td>
</tr>
</table>
<br>
<%
}
%>
<p>
<a href="<%=request.getContextPath()%>/form.jsp">추가메일 등록</a>
</p>
<br>
</body>
</html>
EmaillistDao를 실행시켜서
DB에서 리스트를 가져온다.
그리고 vo 객체에 담아서
알맞은 위치에 넣어주고
화면에 출력해 준다.
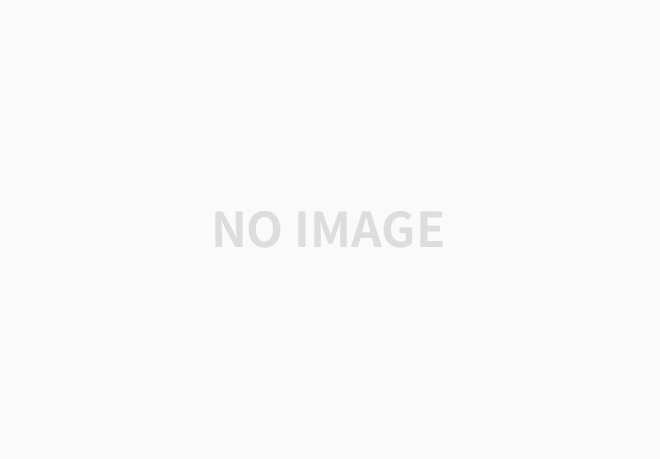
끝.
++++++++++++++++++++++++++++++++++++++++
++++++++++++++++++++++++++++++++++++++++
위의 방식 MVC1으로 만들어본 방명록 이다.
Delete 문을 추가하였기 때문에 위의 것과 쫌 다르다.
위의 과제와 방명록 과제 모두 mvc2 로도 만들 예정이니 비교 할 수 있길 바란다.
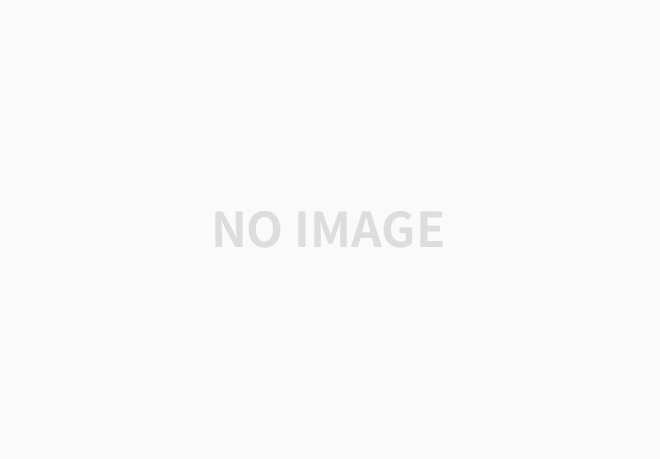
https://github.com/stpn94/servlet-practices/tree/master/guestbook01
GitHub - stpn94/servlet-practices
Contribute to stpn94/servlet-practices development by creating an account on GitHub.
github.com
'Dev > Servlet&JSP' 카테고리의 다른 글
Servlet & JSP 기초 알아가기 2 (0) | 2021.10.12 |
---|---|
Servlet & JSP 기초 와 실습(MVC 2) (0) | 2021.10.08 |
Servlet & JSP 기초 알아가기 1 (0) | 2021.10.07 |